Search results
Deploying Spring Boot applications from a Maven repository to Azure Spring Apps with Azure CLI
1. OVERVIEW
Azure Spring Apps is a platform as a service (PaaS) and one of the services Azure offers for your organization to run Spring Boot applications.
With very little effort, you can provision the Azure Spring Apps infrastructure required to deploy and run Spring Boot Web, RESTful, Batch, etc. applications. This allows your team to spend more time with the applications’ business logic.
This blog post covers provisioning the Azure Spring Apps infrastructure using Azure CLI to deploy a Spring Boot application previously stored in a Maven repository hosted in an Azure Blob Storage container.
Deploying Spring Boot applications to Azure Spring Apps
2. REQUIREMENTS
This tutorial uses Java 11
and Maven 3.8.7
to build and deploy the sample Spring Boot application.
It also uses Azure CLI 2.53.0
to provision the resources to deploy the sample application to Azure Spring Apps.
In case you don’t have Azure CLI, please refer to https://learn.microsoft.com/en-us/cli/azure/install-azure-cli to install it for your OS.
2.1. Azure Spring Apps CLI extension
Let’s install the Azure Spring Apps extension for Azure CLI in case you don’t have it.
az extension add --name spring
az --version
azure-cli 2.53.0
...
Extensions:
spring 1.15.0
...
3. SETUP THE AZURE INFRASTRUCTURE
We’ll run a number of azure-cli
commands to provision the resources to deploy to Azure Spring Apps.
3.1. Login to Azure
az login
...
A browser tab opens with Microsoft Azure Login page. Please login using the Web UI. You can close the tab afterwards.
Once you login, there should be a JSON response in the terminal window like:
[
{
"cloudName": "AzureCloud",
"homeTenantId": "xxxxxxxxxxxxxxx",
"id": "<Your Azure subscription id>"
"name": "<Your Azure subscription name>",
"tenantId": "xxxxxxxxxxxxxxx",
"user": {
...
}
...
}
]
3.2. Set the Azure subscription
az account set -s <Your Azure subscription id>
id
value from the az login command JSON response.
3.3. Create or use a resource group
If you don’t have one yet, you’ll need to create an Azure resource group to include different resources.
az group create --name=<Your resource group name> --location=eastus
I used eastus
for the location, but you can find other Azure locations using azure-cli
:
az account list-locations
and use the name
value from the desired array element as the location for your Azure resource group.
If you would like to use an existing resource group and its location, your could retrieve these values using azure-cli
:
az group list
3.4. Create or use an Azure Spring Apps service instance
Let’s create an Azure Spring Apps service instance if you don’t have one, or you would like to deploy your Spring Boot applications in a new service instance.
az spring create --resource-group <Your resource group name> --name <Your Spring Apps service instance name>
It takes a few minutes to create the Azure Spring Apps service instance. The terminal should display a message along with a JSON-formatted response similar to:
{
"id": "/subscriptions/<Your subscription id>/resourceGroups/<Your resource group name>/providers/Microsoft.AppPlatform/Spring/<Your Spring Apps service instance name>",
"location": "eastus",
"name": "<Your Spring Apps service instance name>",
"properties": {
"fqdn": "<Your Spring Apps service instance name>.azuremicroservices.io",
"networkProfile": {
"outboundIPs": {
"publicIPs": [
"<a.b.c.d>",
"<w.x.y.z>"
],
...
},
"outboundType": "loadBalancer",
...
},
"powerState": "Running",
"provisioningState": "Succeeded",
"serviceId": "<Your Spring Apps service instance id>",
...
},
"resourceGroup": "<Your resource group name>",
...
}
If you would like to use an existing Azure Spring Apps service instance, you could retrieve it with azure-cli
:
az spring list -o table
3.5. Create or use an application in Azure Spring Apps
The last step in provisioning the Azure Spring Apps infrastructure is to create an Azure Spring Apps application where you would deploy your Spring Boot jar application.
az spring app create --resource-group <Your resource group name> --service <Your Spring Apps service instance name> --name <Your Azure Spring Apps application name>
After creating the Azure Spring Apps application, the terminal should display a JSON-formatted response similar to:
{
"id": "/subscriptions/<Your subscription id>/resourceGroups/<Your resource group name>/providers/Microsoft.AppPlatform/Spring/<Your Spring Apps service instance name>/apps/<Your Azure Spring Apps application name>",
"location": "eastus",
"name": "<Your Azure Spring Apps application name>",
...
}
If you would like to use an existing Azure Spring Apps application, you could retrieve it with azure-cli
:
az spring app list --resource-group <Your resource group name> --service <Your Spring Apps service instance name> -o table
4. DEPLOY A SPRING BOOT APPLICATION FROM MAVEN REPOSITORY
A follow-up stage in your build pipeline could be to retrieve the artifact from a Maven repository manager and deploy it to Azure Spring Apps.
4.1. Download the Spring Boot application
Let’s assume the target Spring Boot application to deploy is springboot2-logback-json-1.0.1.jar
. That’s the sample application discussed in JSON-Formatted Logs in Spring Boot applications with Slf4j, Logback and Logstash.
Let’s also assume your organization stores Spring Boot applications, and libraries in a private Maven repository.
Maven repository manager
That’s one place where you would download your applications and deploy them to multiple environments.
production
. These changes invalidate any prior test results.
If your organization stores the artifacts in popular Maven repository managers like Nexus or Artifactory, you could download the target application with this Maven command:
mvn dependency:get -DremoteRepositories=<Your repository URL> -DgroupId=com.asimiotech -DartifactId=springboot2-logback-json -Dversion=1.0.1 -Dpackaging=jar -Dtransitive=false
It will download it to ~/.m2/repository/com/asimiotech/springboot2-logback-json/1.0.1/springboot2-logback-json-1.0.1.jar
I’ll use a different command because the target application is stored in a Maven repository hosted in an Azure Blob Storage Container.
az storage blob download --account-name <Your storage account name> --container-name <Your storage container name> --name com/asimiotech/springboot2-logback-json/1.0.1/springboot2-logback-json-1.0.1.jar --file ./springboot2-logback-json-1.0.1.jar
After downloading the jar application to the current folder, the terminal should output a message and a JSON-formatted response like:
Finished[#############################################################] 100.0000%
{
"container": "<Your storage container name>",
"name": "com/asimiotech/springboot2-logback-json/1.0.1/springboot2-logback-json-1.0.1.jar",
...
}
4.2. Deploy the fat-jar Spring Boot application to Azure Spring Apps
We finally get to deploy the Spring Boot jar application just downloaded.
az spring app deploy --resource-group <Your resource group name> --service <Your Spring Apps service instance name> --name <Your Azure Spring Apps application name> --artifact-path ./springboot2-logback-json-1.0.1.jar
It’ll take a couple of minutes to deploy the Spring Boot jar application to Azure Spring Apps. Once it’s done, you’ll see a few messages about the deployment, the Spring Boot application startup logs followed by a JSON-formatted response:
...
2023-10-25 01:58:16.764Z INFO c.m.applicationinsights.agent - Application Insights Java Agent 3.4.14 started successfully (PID 1, JVM running for 7.019 s)
2023-10-25 01:58:16.768Z INFO c.m.applicationinsights.agent - Java version: 11.0.20.1, vendor: Microsoft, home: /usr/lib/jvm/msopenjdk-11
...
2023-10-25 01:58:27.059 INFO 1 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 1025 (http) with context path ''
2023-10-25 01:58:27.073 INFO 1 --- [ main] com.asimiotech.demo.Application : Started Application in 8.131 seconds (JVM running for 17.328)
...
Spring Boot application deployed to Azure Spring Apps
If it’s an application meant for public access, maybe a Spring Boot UI application written with Thymeleaf and HTMX, you need to use the --assign-endpoint
option in the create application command. Or update the existing Azure Spring Apps application with Azure CLI:
az spring app update --resource-group <Your resource group name> --service <Your Spring Apps service instance name> --name <Your Azure Spring Apps application name> --assign-endpoint
You can now access the UI from the browser, or a public RESTful endpoint following the URL pattern:
https://<Your Spring Apps service instance name>-<Your Azure Spring Apps application name>.azuremicroservices.io
Now that you are able to deploy Spring Boot applications to Azure Spring Apps service, let’s stop the Azure Spring Apps service instance to save costs.
az spring stop --resource-group <Your resource group name> --name <Your Spring Apps service instance name>
5. CONCLUSION
Azure Spring Apps is one of the services Azure provides for your organization to run Spring Boot applications.
Azure Spring Apps service makes Spring Boot applications migration easy.
With a few Azure CLI commands that you can include in your CI/CD build pipeline, you can provision the Azure Spring Apps infrastructure to start deploying Spring Boot applications to.
Once you have the Azure Spring Apps infrastructure in place, you can deploy Spring Boot Web, RESTful, Microservices, or Batch applications that your organization stores in a private Maven repository manager.
6. REFERENCES
NEED HELP?
I provide Consulting Services.ABOUT THE AUTHOR
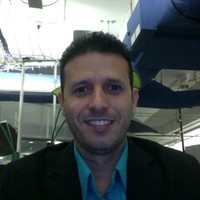